Archive for the ‘Programmierung’ Category.
2015-02-15, 13:36
What I really like about LaTeX is the way one can easily define own commands that get expanded in the text whenever needed. Word has a similar feature, called document variables. Unfortunately these can only be set by VBA macros, there is (according to my knowledge) to way to manage them via GUI. Fortunately Word has another feature which provides a more or less similar functionality with the name „Document properties“.
Here’s a brief tutorial how to define and use a new variable:
1) Open the properties of the document
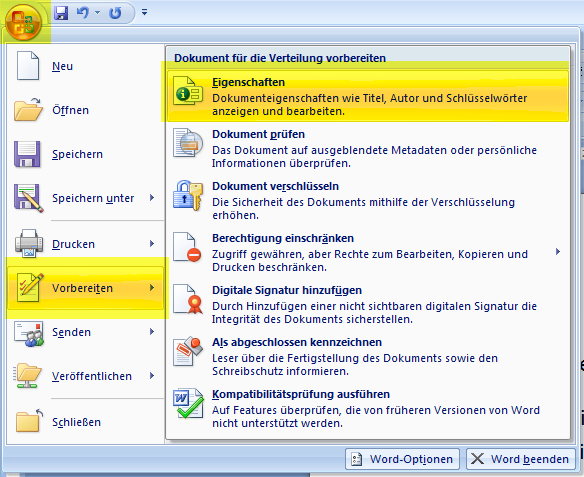
2) Open the extended properties
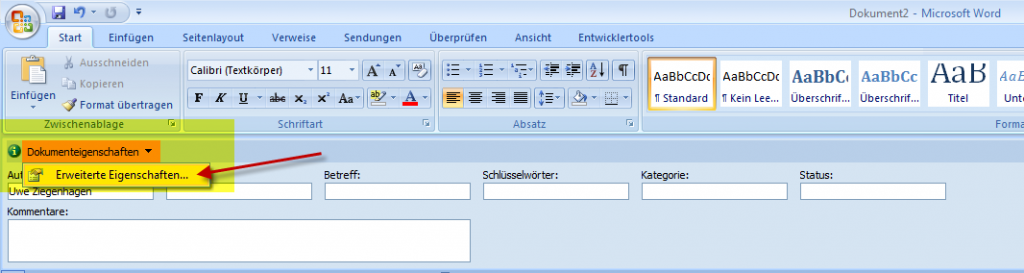
3) Create a new variable, here I used „Projekt“ with the value „MeinProjekt“

4) To use this new variable simply insert a new field:
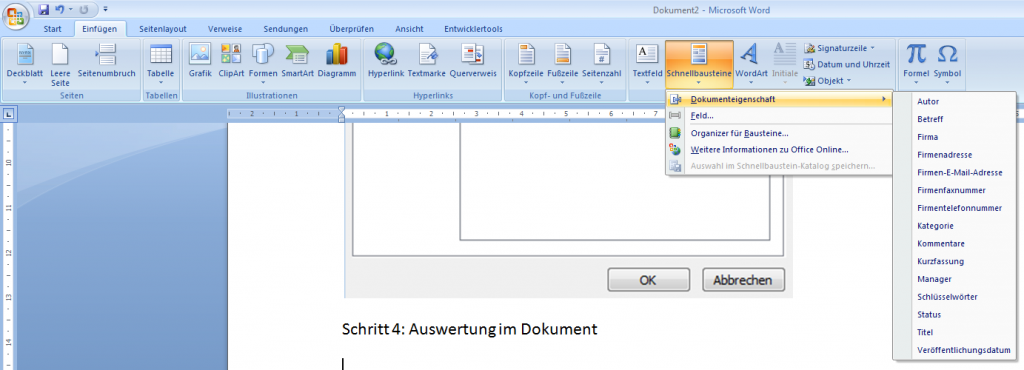
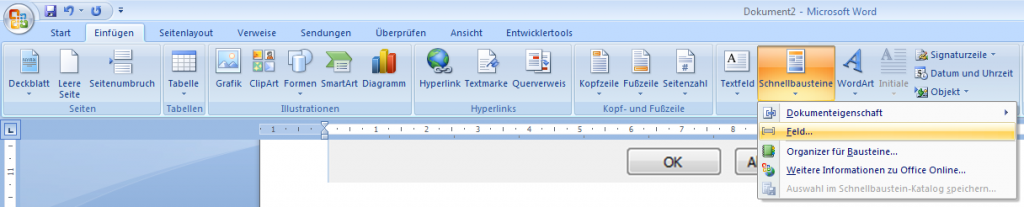
5) and select the corresponding variable

6) The internal syntax is the following (when you press Alt-F9 you get this „internal“ view)

Uwe Ziegenhagen likes LaTeX and Python, sometimes even combined.
Do you like my content and would like to thank me for it? Consider making a small donation to my local fablab, the Dingfabrik Köln. Details on how to donate can be found here Spenden für die Dingfabrik.
More Posts - Website
2015-01-11, 11:47
Die Oster-Formel der Wikipedia kann man recht einfach in einem Python-Algorithmus nutzen:
import datetime
def calcEaster(jahr):
a = jahr % 19
b = jahr % 4
c = jahr % 7
k = jahr / 100
p = (8 * k + 13) / 25
q = k / 4
M = (15 + k - p - q) % 30
d = (19 * a + M) % 30
N = (4 + k - q) % 7
e = (2 * b + 4 * c + 6 * d + N) % 7
Ostermontag = (22 + d + e) - 1
start = datetime.datetime.strptime("01.03." + str(jahr), "%d.%m.%Y")
easter = start + datetime.timedelta(days=round(Ostermontag))
return easter.strftime('%d.%m.%Y')
for i in range(2014,2050):
print(calcEaster(i)) |
import datetime
def calcEaster(jahr):
a = jahr % 19
b = jahr % 4
c = jahr % 7
k = jahr / 100
p = (8 * k + 13) / 25
q = k / 4
M = (15 + k - p - q) % 30
d = (19 * a + M) % 30
N = (4 + k - q) % 7
e = (2 * b + 4 * c + 6 * d + N) % 7
Ostermontag = (22 + d + e) - 1
start = datetime.datetime.strptime("01.03." + str(jahr), "%d.%m.%Y")
easter = start + datetime.timedelta(days=round(Ostermontag))
return easter.strftime('%d.%m.%Y')
for i in range(2014,2050):
print(calcEaster(i))
Ergänzung: Das Osterparadoxon ist nicht berücksichtigt!
Uwe Ziegenhagen likes LaTeX and Python, sometimes even combined.
Do you like my content and would like to thank me for it? Consider making a small donation to my local fablab, the Dingfabrik Köln. Details on how to donate can be found here Spenden für die Dingfabrik.
More Posts - Website
2014-12-14, 18:53
Today I had to TeX a longer file with numerous \cite[somestuff]{citekey}
sequences, of which some had hard-to-spot errors (some ‚]‘ were missing). The following Python script checks if each \cite
command has matching '[]'
and '{}'
sequences. I’ll probably extend this script to accept simple \cite{citekey}
sequences that have no optional parameter.
#!/usr/bin/python
import re
with open('filetocheck.tex') as f:
content = f.readlines()
index = 0
for a in content:
index = index + 1
if "cite" in a: # check only lines that contain 'cite'
matches = re.search(r'(.*)\[(.*)\]\{(.*)\}',a) # search for <sometext>[<sometext>]{<sometext>}
if not matches:
print (">>> Fail in row" , str(index), a) |
#!/usr/bin/python
import re
with open('filetocheck.tex') as f:
content = f.readlines()
index = 0
for a in content:
index = index + 1
if "cite" in a: # check only lines that contain 'cite'
matches = re.search(r'(.*)\[(.*)\]\{(.*)\}',a) # search for <sometext>[<sometext>]{<sometext>}
if not matches:
print (">>> Fail in row" , str(index), a)
Uwe Ziegenhagen likes LaTeX and Python, sometimes even combined.
Do you like my content and would like to thank me for it? Consider making a small donation to my local fablab, the Dingfabrik Köln. Details on how to donate can be found here Spenden für die Dingfabrik.
More Posts - Website
2014-11-15, 00:23
Here’s the probably last part of this series, the missing piece showing the calculation of MD5 files with Python:
# http://www.pythoncentral.io/hashing-files-with-python/
import hashlib
import os
import codecs
hasher = hashlib.md5()
def calcHash(filename):
print("Filename: " + filename)
with open(filename, 'rb') as afile:
buf = afile.read()
hasher.update(buf)
print("Calculated: " + hasher.hexdigest())
md5file = (os.path.splitext(filename)[0]) + ".md5"
writeMD5 = codecs.open(md5file, "wb", "utf-8-sig")
writeMD5.write(hasher.hexdigest())
calcHash("e:/arara.log") |
# http://www.pythoncentral.io/hashing-files-with-python/
import hashlib
import os
import codecs
hasher = hashlib.md5()
def calcHash(filename):
print("Filename: " + filename)
with open(filename, 'rb') as afile:
buf = afile.read()
hasher.update(buf)
print("Calculated: " + hasher.hexdigest())
md5file = (os.path.splitext(filename)[0]) + ".md5"
writeMD5 = codecs.open(md5file, "wb", "utf-8-sig")
writeMD5.write(hasher.hexdigest())
calcHash("e:/arara.log")
Uwe Ziegenhagen likes LaTeX and Python, sometimes even combined.
Do you like my content and would like to thank me for it? Consider making a small donation to my local fablab, the Dingfabrik Köln. Details on how to donate can be found here Spenden für die Dingfabrik.
More Posts - Website
2014-11-07, 19:23
Today I needed to split an integer (representing a bitmask) into its components, each component in a separate column. The following code from http://www.sqlservercentral.com/Forums/Topic1101943-392-1.aspx did the trick.
SELECT
N,
SIGN(N & 1) AS Bit1,
SIGN(N & 2) AS Bit2,
SIGN(N & 4) AS Bit3,
SIGN(N & 8) AS Bit4,
SIGN(N & 16) AS Bit5,
SIGN(N & 32) AS Bit6,
SIGN(N & 64) AS Bit7,
SIGN(N & 128) AS Bit8
FROM (
SELECT 511
) TestData(N) |
SELECT
N,
SIGN(N & 1) AS Bit1,
SIGN(N & 2) AS Bit2,
SIGN(N & 4) AS Bit3,
SIGN(N & 8) AS Bit4,
SIGN(N & 16) AS Bit5,
SIGN(N & 32) AS Bit6,
SIGN(N & 64) AS Bit7,
SIGN(N & 128) AS Bit8
FROM (
SELECT 511
) TestData(N)
Uwe Ziegenhagen likes LaTeX and Python, sometimes even combined.
Do you like my content and would like to thank me for it? Consider making a small donation to my local fablab, the Dingfabrik Köln. Details on how to donate can be found here Spenden für die Dingfabrik.
More Posts - Website
Category:
Programmierung,
SQL |
Kommentare deaktiviert für SQL: Splitting a Bitmask into separate columns
2014-11-06, 22:19
Here some Python code to calculate the MD5 hash for a given file:
# from http://www.pythoncentral.io/hashing-files-with-python/
import hashlib
hasher = hashlib.md5()
with open('e:/Mappe1.xlsx', 'rb') as afile:
buf = afile.read()
hasher.update(buf)
print(hasher.hexdigest()) |
# from http://www.pythoncentral.io/hashing-files-with-python/
import hashlib
hasher = hashlib.md5()
with open('e:/Mappe1.xlsx', 'rb') as afile:
buf = afile.read()
hasher.update(buf)
print(hasher.hexdigest())
Here the final script that provides the same functionality as the Powershell script I posted earlier today:
# http://www.pythoncentral.io/hashing-files-with-python/
import hashlib
import os
import codecs
hasher = hashlib.md5()
def calcHash(filename):
print("Filename: " + filename)
with open(filename, 'rb') as afile:
buf = afile.read()
hasher.update(buf)
print("Calculated: " + hasher.hexdigest())
md5file = (os.path.splitext(filename)[0]) + ".md5"
givenMD5 = codecs.open(md5file, "r", "utf-8-sig")
print("Provided: " + str(givenMD5.read()).lower())
calcHash("e:/Mappe1.xlsx") |
# http://www.pythoncentral.io/hashing-files-with-python/
import hashlib
import os
import codecs
hasher = hashlib.md5()
def calcHash(filename):
print("Filename: " + filename)
with open(filename, 'rb') as afile:
buf = afile.read()
hasher.update(buf)
print("Calculated: " + hasher.hexdigest())
md5file = (os.path.splitext(filename)[0]) + ".md5"
givenMD5 = codecs.open(md5file, "r", "utf-8-sig")
print("Provided: " + str(givenMD5.read()).lower())
calcHash("e:/Mappe1.xlsx")
Uwe Ziegenhagen likes LaTeX and Python, sometimes even combined.
Do you like my content and would like to thank me for it? Consider making a small donation to my local fablab, the Dingfabrik Köln. Details on how to donate can be found here Spenden für die Dingfabrik.
More Posts - Website
2014-11-06, 20:56
Here’s some code to a) calculate a MD5 hash for a given file and b) to compare the hash with a MD5 provided in an external file. The scripts expects the MD5 file to have the same name, but MD5 extension. Credit goes to the friendly souls providing the original code, I just copied and pasted everything together.
The comparison is just visual, both MD5 hashes — the calculated and the provided one — are printed on the screen, it’s easily to extend it for an automated comparison.
# Reference: http://onlinemd5.com/
### Step A: Get the name of the file
# http://blogs.technet.com/b/heyscriptingguy/archive/2009/09/01/hey-scripting-guy-september-1.aspx
Function Get-FileName($initialDirectory)
{
[System.Reflection.Assembly]::LoadWithPartialName("System.windows.forms") |
Out-Null
$OpenFileDialog = New-Object System.Windows.Forms.OpenFileDialog
$OpenFileDialog.initialDirectory = $initialDirectory
$OpenFileDialog.filter = "All files (*.*)| *.*"
$OpenFileDialog.ShowDialog() | Out-Null
$OpenFileDialog.filename
} #end function Get-FileName
# *** Entry Point to Script ***
$file = Get-FileName -initialDirectory "H:\MD5"
### Step B: Create a file handle from this string and calculate the MD5 sum
$filehandle = Get-ChildItem $file
# write-host "file: " $file
# write-host "DirectoryName: " $filehandle.DirectoryName
write-host "Name: " $filehandle.Name
# write-host "pure Name: " $filehandle.BaseName
# http://stackoverflow.com/questions/10521061/how-to-get-an-md5-checksum-in-powershell
$md5 = New-Object -TypeName System.Security.Cryptography.MD5CryptoServiceProvider
$hash = [System.BitConverter]::ToString($md5.ComputeHash([System.IO.File]::ReadAllBytes($filehandle))).ToLower().Replace("-","")
write-host "Calculated Hashsum: " $hash
### Step C: Get the MD5 sum from the MD5 sum file matching the other filename
$md5handle = Get-ChildItem $($filehandle.DirectoryName + "\" + $filehandle.BaseName + ".md5")
$md5sum = [IO.File]::ReadAllText($md5handle)
write-host "Provided MD5 " $md5sum |
# Reference: http://onlinemd5.com/
### Step A: Get the name of the file
# http://blogs.technet.com/b/heyscriptingguy/archive/2009/09/01/hey-scripting-guy-september-1.aspx
Function Get-FileName($initialDirectory)
{
[System.Reflection.Assembly]::LoadWithPartialName("System.windows.forms") |
Out-Null
$OpenFileDialog = New-Object System.Windows.Forms.OpenFileDialog
$OpenFileDialog.initialDirectory = $initialDirectory
$OpenFileDialog.filter = "All files (*.*)| *.*"
$OpenFileDialog.ShowDialog() | Out-Null
$OpenFileDialog.filename
} #end function Get-FileName
# *** Entry Point to Script ***
$file = Get-FileName -initialDirectory "H:\MD5"
### Step B: Create a file handle from this string and calculate the MD5 sum
$filehandle = Get-ChildItem $file
# write-host "file: " $file
# write-host "DirectoryName: " $filehandle.DirectoryName
write-host "Name: " $filehandle.Name
# write-host "pure Name: " $filehandle.BaseName
# http://stackoverflow.com/questions/10521061/how-to-get-an-md5-checksum-in-powershell
$md5 = New-Object -TypeName System.Security.Cryptography.MD5CryptoServiceProvider
$hash = [System.BitConverter]::ToString($md5.ComputeHash([System.IO.File]::ReadAllBytes($filehandle))).ToLower().Replace("-","")
write-host "Calculated Hashsum: " $hash
### Step C: Get the MD5 sum from the MD5 sum file matching the other filename
$md5handle = Get-ChildItem $($filehandle.DirectoryName + "\" + $filehandle.BaseName + ".md5")
$md5sum = [IO.File]::ReadAllText($md5handle)
write-host "Provided MD5 " $md5sum
Uwe Ziegenhagen likes LaTeX and Python, sometimes even combined.
Do you like my content and would like to thank me for it? Consider making a small donation to my local fablab, the Dingfabrik Köln. Details on how to donate can be found here Spenden für die Dingfabrik.
More Posts - Website
Schlagwörter:
Powershell,
MD5 Category:
Powershell |
Kommentare deaktiviert für Using Powershell to calculate/compare MD5 hashes
2014-07-13, 16:41
Vor kurzem hatte ich eine kleine Herausforderung in Excel: Beträge in Spalte C mussten mit negativem Vorzeichen versehen werden, in Abhängigkeit einer Zahl in Spalte B (gelb markiert im Bild). Lösen lässt sich dies per verschachtelter WENN()
Funktion, bei vielen Verschachtelungen wird die Formel aber schnell unübersichtlich und fehleranfällig. Deutlich einfacher und übersichtlicher geht es über eine Mischung aus VERGLEICH()
und ISTNV()
, die ich bei stackexchange gefunden hatte.
=WENN(ISTNV(VERGLEICH([@Unterkonto];unterkonten;0));[@Amount];-1*[@Amount])
- für die Zahlen, die den Vorzeichenwechsel auslösen, habe ich einen benannten Bereich ‚unterkonten‘ festgelegt
VERGLEICH()
schaut, ob ein Wert (der erste Parameter) in einer Liste (zweiter Parameter) vorhanden ist. Wird ein Wert nicht gefunden, wird #NV
zurückgeliefert. Der zweite Parameter kann auch ein benannter Bereich sein.
- Um die
#NV
Werte zu behandeln, prüfen wir mit ISTNV()
die Rückgabe der VERGLEICH()
Funktion
- Die Rückgabe von
ISTNV()
wird dann per WENN()
geprüft. Wenn ISTNV()
WAHR zurückliefert, dann hat VERGLEICH()
das Konto nicht in der Liste gefunden, sonst ist der Werte aus Spalte „Amount“ mit -1 zu multiplizieren.
Download der Excel-Datei
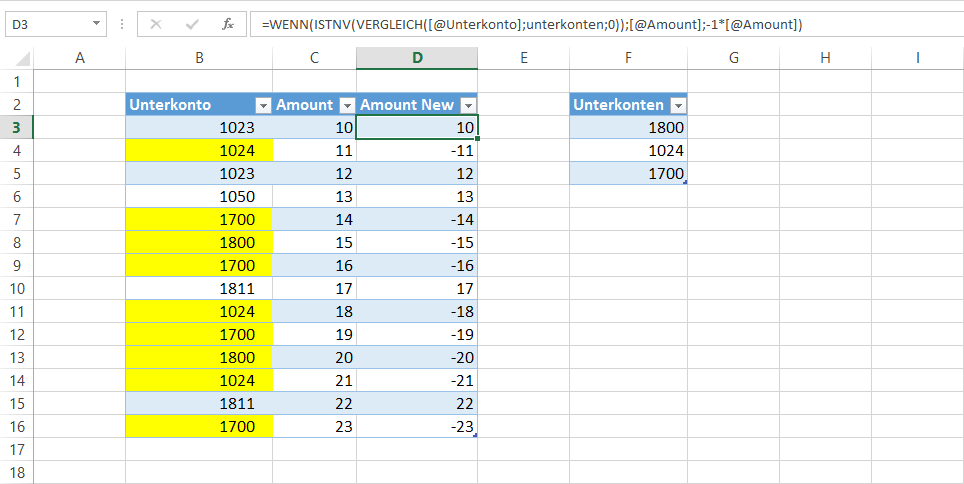
Uwe Ziegenhagen likes LaTeX and Python, sometimes even combined.
Do you like my content and would like to thank me for it? Consider making a small donation to my local fablab, the Dingfabrik Köln. Details on how to donate can be found here Spenden für die Dingfabrik.
More Posts - Website
2014-06-15, 13:24
Here’s a simple example how to create a menu with Autohotkey, in this case it allows the user to select one of the LaTeX standard environments itemize, enumerate or description when Alt-y
is pressed.
!y::
Gui, 1:Destroy
Gui, Add, Text,, Please enter the environment:
Gui, Add, DDL, vList, itemize||enumerate|description
Gui, Add, Button, Default, Input
Gui, Show
return
ButtonInput:
Gui, Submit, NoHide
Gui, 1:Destroy
Send %List%
return
Uwe Ziegenhagen likes LaTeX and Python, sometimes even combined.
Do you like my content and would like to thank me for it? Consider making a small donation to my local fablab, the Dingfabrik Köln. Details on how to donate can be found here Spenden für die Dingfabrik.
More Posts - Website
2014-02-19, 21:26
Here’s a short code sample how to list a remote directory via FTP.
from ftplib import FTP
ftp = FTP('server')
ftp.connect()
ftp.login('user','password')
ftp.cwd('folder')
ftp.dir()
ftp.quit() |
from ftplib import FTP
ftp = FTP('server')
ftp.connect()
ftp.login('user','password')
ftp.cwd('folder')
ftp.dir()
ftp.quit()
Hier noch der passende Code für den Upload von Dateien:
import ftplib
import os
def upload(ftp, file):
ext = os.path.splitext(file)[1]
print("Putting ", file)
if ext in (".txt", ".htm", ".html" , ".tex"):
ftp.storlines('STOR ' + file, open(file,'rb'))
else:
ftp.storbinary('STOR ' + file, open(file,'rb'))
ftp = ftplib.FTP("remoteserver")
ftp.login("user", "password")
upload(ftp, "index.html") |
import ftplib
import os
def upload(ftp, file):
ext = os.path.splitext(file)[1]
print("Putting ", file)
if ext in (".txt", ".htm", ".html" , ".tex"):
ftp.storlines('STOR ' + file, open(file,'rb'))
else:
ftp.storbinary('STOR ' + file, open(file,'rb'))
ftp = ftplib.FTP("remoteserver")
ftp.login("user", "password")
upload(ftp, "index.html")
Uwe Ziegenhagen likes LaTeX and Python, sometimes even combined.
Do you like my content and would like to thank me for it? Consider making a small donation to my local fablab, the Dingfabrik Köln. Details on how to donate can be found here Spenden für die Dingfabrik.
More Posts - Website